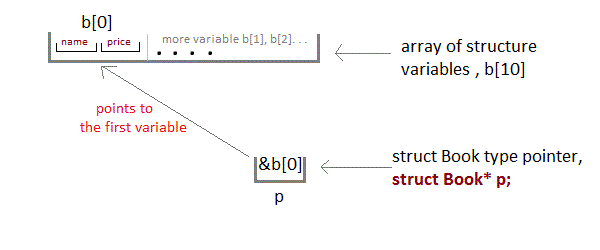
Pointer to Structure
Like we have array of integers, array of pointer etc, we can also have array of structure variables. And to make the use of array of structure variables efficient, we use pointers of structure type. We can also have pointer to a single structure variable, but it is mostly used with array of structure variables.
struct Book
{
char name[10];
int price;
}
int main()
{
...