Pointer and Arrays
When an array is declared, compiler allocates sufficient amount of memory to contain all the elements of the array. Base address which gives location of the first element is also allocated by the compiler.
Suppose we declare an array arr,
int arr[5]={ 1, 2, 3, 4, 5 };
Assuming that the base address of arr is 1000 and each integer requires two byte, the five element will be stored as follows
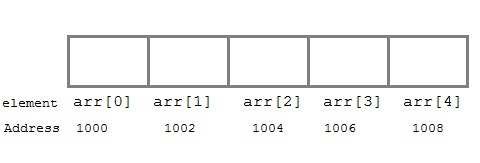
Here variable arr will give the base address, which is a constant pointer pointing to the element, arr[0]. Therefore arr is containing the address of arr[0] i.e 1000.
arr is equal to &arr[0] // by default
We can declare a pointer of type
int
to point to the array arr.int *p;
p = arr;
or p = &arr[0]; //both the statements are equivalent.
Now we can access every element of array arr using p++ to move from one element to another.
NOTE : You cannot decrement a pointer once incremented.
p--
won't work.Pointer to Array
As studied above, we can use a pointer to point to an Array, and then we can use that pointer to access the array. Lets have an example,
int i; int a[5] = {1, 2, 3, 4, 5}; int *p = a; // same as int*p = &a[0] for (i=0; i<5; i++) { printf("%d", *p); p++; }
In the above program, the pointer *p will print all the values stored in the array one by one. We can also use the Base address (a in above case) to act as pointer and print all the values.
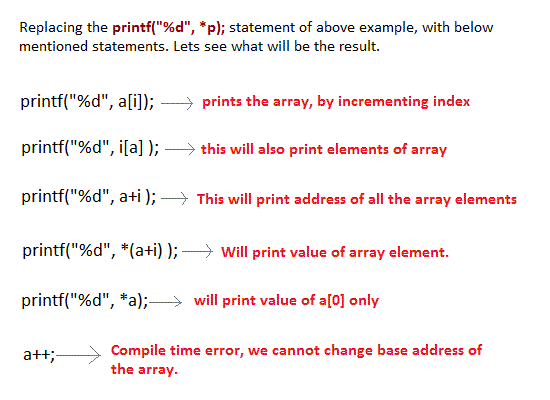
Pointer to Multidimensional Array
A multidimensional array is of form,
a[i][j]
. Lets see how we can make a pointer point to such an array. As we know now, name of the array gives its base address. In a[i][j]
, a will give the base address of this array, even a+0+0
will also give the base address, that is the address of a[0][0] element.
Here is the generalized form for using pointer with multidimensional arrays.
*(*(ptr + i) + j)
is same as
a[i][j]
Pointer and Character strings
Pointer can also be used to create strings. Pointer variables of char type are treated as string.
char *str = "Hello";
This creates a string and stores its address in the pointer variable str. The pointer str now points to the first character of the string "Hello". Another important thing to note that string created using char pointer can be assigned a value at runtime.
char *str; str = "hello"; //thi is Legal
The content of the string can be printed using
printf()
and puts()
.printf("%s", str); puts(str);
Notice that str is pointer to the string, it is also name of the string. Therefore we do not need to use indirection operator
*
.Array of Pointers
We can also have array of pointers. Pointers are very helpful in handling character array with rows of varying length.
char *name[3]={ "Adam", "chris", "Deniel" }; //Now see same array without using pointer char name[3][20]= { "Adam", "chris", "Deniel" };
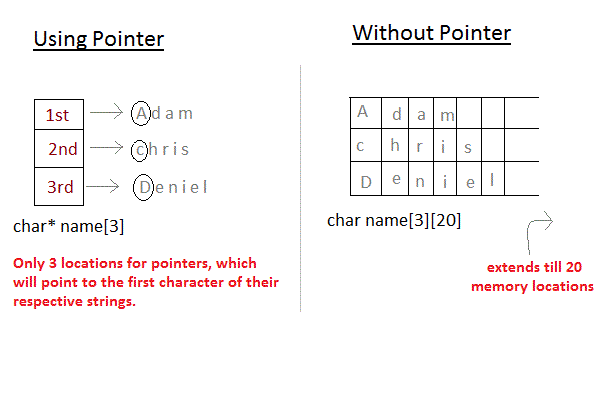
In the second approach memory wastage is more, hence it is prefered to use pointer in such cases.
No comments:
Post a Comment